6. Pushbuttons and Events¶
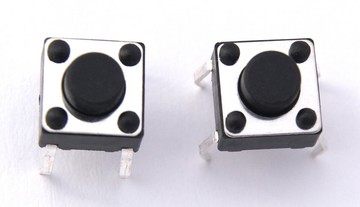
Goals¶
- Read the events associated with a button.
- Program Arduino to respond to pushbutton events.
Pushbutton related events¶

The keyEvents()
function¶
-
int
keyEvents
(int keyNum, int event)¶ This function returns the value of the events that occur in a button. The events that the function can return are the following:
Event Meaning KEY_PRESSED_TIME1 The push button has been pressed for 0.02 seconds KEY_PRESSED_TIME2 The push button has been pressed for 0.5 seconds KEY_PRESSED_TIME3 Push button has been pressed for 2.0 seconds KEY_RELEASED The button has been released These time values are the default values after starting the board and can be changed in the settings.
keyNum
: número del 1 al 6 que representa al pulsador del que se solicita su valor. El valor 0 representa a todos los pulsadores juntos.
Turn on a led when a button is pressed for a certain time¶
In this example, a led will light after its push button is pressed for more than half a second.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | // Enciende el led D1 cuando se presiona el pulsador 1
// más de medio segundo
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Si (pulsador 1 es presionado-medio-segundo)
if (pc.keyEvents(1, KEY_PRESSED_TIME2))
// Enciende el led D1
pc.ledWrite(1, LED_ON);
}
|
The example can be easily modified to turn on after a two second press.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | // Enciende el led D1 cuando se presione el pulsador 1
// más de dos segundos
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Si (pulsador 1 es presionado-dos-segundos)
if (pc.keyEvents(1, KEY_PRESSED_TIME3))
// Enciende el led D1
pc.ledWrite(1, LED_ON);
}
|
Multiple functions in one button¶
This example is a bit more complex and demonstrates the ability of events to give more than one meaning to a single push button. Thanks to this capability, a single push button will be able to perform many functions on its own.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | // Tres funciones en el pulsador 1:
// Al presionar poco tiempo, se enciende el led D1
// Al presionar más de medio segundo, parpadea el led D1
// Al presionar más de dos segundos, se apaga el led D1
#include <Wire.h>
#include <PC42.h>
void setup() {
pc.begin(); // Inicializar el módulo PC42
}
void loop() {
// Si (pulsador 1 es recién-presionado)
if (pc.keyEvents(1, KEY_PRESSED_TIME1))
// Enciende el led D1
pc.ledWrite(1, LED_ON);
// Si (pulsador 1 es presionado-medio-segundo)
if (pc.keyEvents(1, KEY_PRESSED_TIME2))
// Parpadea el led D1 rápido
pc.ledBlink(1, 200, 200);
// Si (pulsador 1 es presionado-dos-segundos)
if (pc.keyEvents(1, KEY_PRESSED_TIME3))
// Apaga el led D1
pc.ledWrite(1, LED_OFF);
}
|
Exercises¶
Program the code needed to solve the following problems.
The following program turns on led D1 when button D1 is pressed and turns off led D1 when button 1 is pressed again. A variable is used to store the state of led D1. It is requested to modify the program so that led D2 also turns on and off with button 2.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
// Enciende y apaga el led D1 con el pulsador 1 #include <Wire.h> #include <PC42.h> int on_off_1; void setup() { pc.begin(); // Inicializar el módulo PC42 on_off_1 = 0; // El led D1 comienza apagado } void loop() { pc.ledWrite(1, on_off_1); // Enciende o apaga el led D1 // Si (evento de pulsador 1 es igual a pulsado) if (pc.keyEvents(1, KEY_PRESSED_TIME1)) { // Cambia el estado de encendido <--> apagado on_off_1 = 1 - on_off_1; } }
The following program moves a led to the right when button 2 is pressed. Modify the program so that the led moves to the left when button 1 is pressed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
// Mueve la luz a la derecha al presionar el pulsador 2 #include <Wire.h> #include <PC42.h> int led; void setup() { pc.begin(); // Inicializar el módulo PC42 led = 1; // Enciende primero el led D1 } void loop() { // Enciende el led actual pc.ledWrite(led, LED_ON); // Si se presiona la tecla derecha if (pc.keyEvents(KEY_RIGHT, KEY_PRESSED_TIME1)) { pc.ledWrite(led, LED_OFF); // Apaga el led actual led = led + 1; // Mover el led a la derecha if (led > 6) // Si se pasa por la derecha led = 1; // volver al inicio } }
Modify the previous program so that led D1 lights up and the rest of the leds go off when pressing button 6
KEY_BACK
for two seconds.